jsp: useBean
Introduction:

Introduction:
1. If we are using scriptlet and expression there is no clear separation of presentation and business logic.
2. The person who is writing Jsp should have compulsory the knowledge of both Java and html which may not possible always.
3. It reduces the readability and maintainability.
4. It reduces reusability of the code also.
We can recover these problems by encapsulating business logic inside a Java Bean class.
Syntax:
<jsp:useBean id="objectName" class="package.class" /> |
<jsp: useBean>
We can use this standard action to make java bean object available to the Jsp.
There are two forms of <jsp: useBean>
1. Without Body
<jsp:useBean id="b" class="com.myjavahub.SimpleBean"/> |
2. With Body
<jsp:useBean id="b" class="com.myjavahub.SimpleBean"> // code … </jsp:useBean> |
Note : The main objective of body is to perform initialization for the newly created bean object.If the bean object is already available then <jsp:useBean> tag won’t create any new object it will use existing object only In this case body won’t be executed.
Advantage of JavaBean :
1. Separation of presentation & business logic
Business logic is available in Bean Class and presentation logic available in Jsp. It improves readability and maintainability of the code.
2. Separation of responsibilities
Java developer can concentrate on business logic where as html page designer can concentrate on presentation logic. As both can work simultaneously, we can reduce development time.
3. It promotes reusability of the code.
Where ever square It functionality is required we can use the same Bean without rewiring.
We can purchase a bean for file uploading and we can start uploading of files within a few minutes.
Example:
SimpleBean.java
package com.myjavahub; public class SimpleBean { private String uname ; private String password ; private String mail ; private String mobileno; public String getUname() { return uname; } public void setUname(String uname) { this.uname = uname; } public String getPassword() { return password; } public void setPassword(String password) { this.password = password; } public String getMail() { return mail; } public void setMail(String mail) { this.mail = mail; } public String getMobileno() { return mobileno; } public void setMobileno(String mobileno) { this.mobileno = mobileno; } } |
test.jsp
<jsp:useBean id="b" class="com.myjavahub.SimpleBean"></jsp:useBean> <% b.setMail("myjavahub@gmail.com"); b.setMobileno("9999999999"); b.setPassword("myjavahub"); b.setUname("myjavahub"); %> <table border=2> <tr><td><h2>property</h2></td><td><h2>value</h2></td></tr> <tr><td><h2>User Name</h2></td><td><h2><%= b.getUname()%></h2></td></tr> <tr><td><h2>Password</h2></td><td><h2><%= b.getPassword()%></h2></td></tr> <tr><td><h2>Mail Id</h2></td><td><h2><%= b.getMail()%></h2></td></tr> <tr><td><h2>Mobile Num</h2></td><td><h2><%= b.getMobileno()%></h2></td></tr> </table> |
Output: http://localhost:8080/Scwcd_jsp/test.jsp

What is the java bean ?
1. A Java Bean is a simple java class.
2. The bean class should compulsory contain public no argument constructor otherwise
<jsp: useBean> tag won’t work .
3. For every property public getter and setter methods are required otherwise
<jsp: getProperty> and jsp: setProperty> won’t work properly.
Attributes of <jsp: useBean>
<jsp:useBean> tag can contain the following five attributes.
1. id
2. class
3. type
4. Scope
5. beanName
1. id
It represents the name of the reference variable of the bean object. And by using this reference variable only we can access bean in rest of the jsp.This attribute is mandatory attribute.
2. class Attribute
It specifies fully qualified Name of the Bean class.For this class only Jsp engine performs instantiation and class should be concrete class, Abstract classes and interfaces are not allowed for class attribute.Class attribute is optional but we have to specify either class attribute or type attribute is mandatory
3. type Attribute
This attribute can be used to specify the type of the reference variable.The value of the type attribute can be Concrete class (or) Abstract class (or) Interface.class attribute is optional but we have to specify either class attribute or type attribute is mandatory
4.scope Attribute
This attribute specifies jsp engine in which scope has to search for the required bean object.if the java bean object is not available in that scope jsp engine create a new bean and object and store in specified scope for the future purpose.The allowed values for the scope attribute are
§ page
§ request
§ Session
§ application
Note: scope attribute is optional and default scope is page.
<% @ page session = “false”%> <jsp: UseBean id= “c” class= “pack1.calculatorBean” scope= “session”/> |
Error:-Illegal for Use Bean to use session scope when Jsp page declares (via page directive) that is does not participate in sessions.
5. Bean Name
There may be a chance of using serialized bean object from local file system.
In that case we have to use bean name attribute.
Note :If we are using only type attribute without class then compulsory the specified type bean object should be available otherwise Jsp engine won’t create any new bean object and raises instantiation exception.
EX : <jsp: useBean id= “c” type= “java.lang.Runnable” scope= “session”/>
Jsp engine searches for Runnable type object with “c” name in session scope. If it is not available then it raises runtime exception.
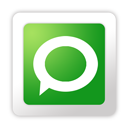
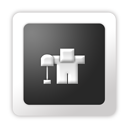
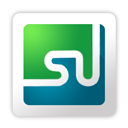
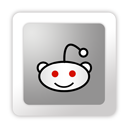
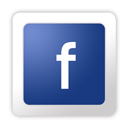
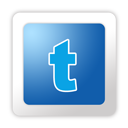
No comments:
Post a Comment