Introduction:
Example:

1. For each request the JSP engine creates a new object to represents that request called request object.
2. The request object is an instance of class javax.servlet.http.HttpServletRequest .
3. The request object contains all information about the HTTP header, query string, cookies.
4. Be noted that request object only available in a scope of the current request. It is re-created each time new request is made.
Methods of request Object:
There are numerous methods available for request Object. Some of them are:
1.getCookies() | The getCookies() method of request object returns all cookies sent with the request information by the client. The cookies are returned as an array of Cookie Objects. |
2.getHeader(String name) | The method getHeader(String name) of request object is used to return the value of the requested header. |
3.getHeaderNames() | The method getHeaderNames() of request object returns all the header names in the request. |
4.getAttribute(String name) | The method getAttribute() of request object is used to return the value of the attribute. The getAttribute() method returns the objects associated with the attribute. |
5.getAttributeNames() | The method getAttribute() of request object is used to return the object associated with the particular given attribute. |
6.getMethod() | The getMethod() of request object is used to return the methods GET, POST, or PUT corresponding to the requested HTTP method used. |
7.getParameter(String name) | getParameter() method of request object is used to return the value of a requested parameter. |
8.getParameterNames() | The getParameterNames() method of request object is used to return the names of the parameters given in the current request. |
9.getParameterValues(String name) | The getParameter(String name) method of request object was used to return the value of a requested given parameter. |
10.getQueryString() | The getQueryString() method of request object is used to return the query string from the request. |
11.getRequestURI() | The getRequestURI() method of request object is used for returning the URL of the current JSP page. |
12.getServletPath() | The getServletPath() method of request object is used to return the part of request URL that calls the servlet. |
13.setAttribute(String,Object) | The setAttribute method of request object is used to set object to the named attribute. |
14.removeAttribute(String) | The removeAttribute method of request object is used to remove the object bound with specified name from the corresponding session. |
Example:
Test.jsp:
<%@ page import = " java.util.* " %> <html> <head> <title>Getting a header in Jsp</title> </head> <body> <% Enumeration enumeration = request.getHeaderNames(); out.print("<table border= 2>"); while (enumeration.hasMoreElements()) { String string = (String)enumeration.nextElement(); out.println("<tr><td><h3>" +string +":</h3></td><td><h3> " + request.getHeader(string)+ "</h3></td></tr>"); } out.print("</table>"); %> </body> </html> |
Output:

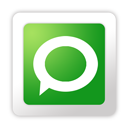
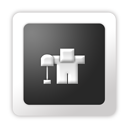
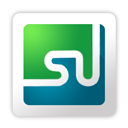
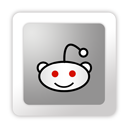
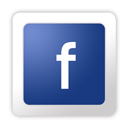
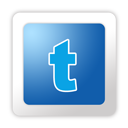
No comments:
Post a Comment